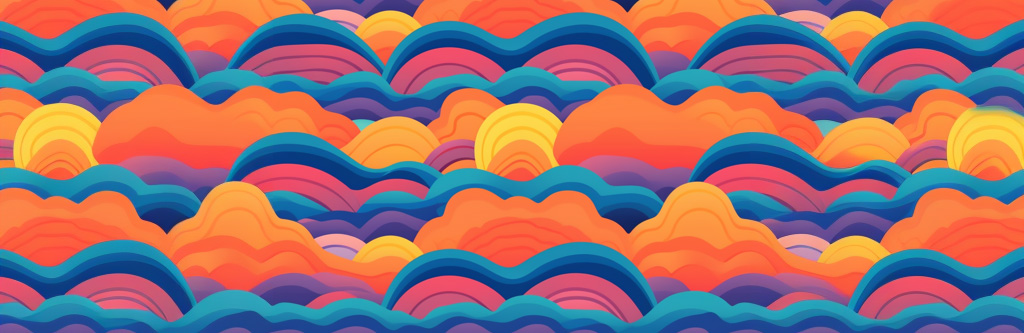
In web development, the concept of “live reloading” refers to automatically reloading the browser whenever you make changes to your code. This feature significantly improves development efficiency by eliminating the need to manually refresh the browser each time you make a small adjustment, particularly to your CSS or PHP files. For WordPress theme development, live reloading is especially useful, as it allows you to see your changes instantly without interrupting your workflow.
In this post, I’ll walk through how to set up live reloading for your WordPress theme development environment using Gulp, BrowserSync, and Sass. By the end, you’ll have a system in place that automatically refreshes the browser whenever you modify your SCSS, CSS, or PHP files.
Installation
To get live reloading working, we need to set up a few tools within the theme’s directory. We’ll use Gulp (a task runner), BrowserSync (which will handle the automatic browser refresh), and Sass (for compiling your SCSS into CSS).
Before getting started, make sure you have Node.js installed on your system. Node.js will provide access to npm, which we’ll use to install the necessary packages.
1. Navigate to Your Theme Directory
Open your terminal and navigate to the root directory of your WordPress theme:
cd /path-to-your-wordpress/wp-content/themes/your-theme
2. Initialize a New npm Project
Inside the theme directory, initialize a new npm project by running:
npm init -y
3. Install the Required Packages
Now, we’ll install Gulp, BrowserSync, and Sass by running the following command:
npm install gulp browser-sync sass gulp-sass --save-dev
This will install Gulp to automate tasks, BrowserSync for live reloading, and Gulp-Sass to compile SCSS files into CSS. The --save-dev
flag ensures these are saved as development dependencies.
If you don’t have Gulp installed globally, you’ll also need to run:
npm install --global gulp-cli
Setting Up the Gulp File
Now that we’ve installed the necessary packages, the next step is to create a gulpfile.js
in your theme folder. This file will contain the tasks Gulp will perform, such as compiling Sass and reloading the browser on file changes.
In your theme directory, create a new file called gulpfile.js
. This is where you’ll write the Gulp tasks that handle live reloading and compiling Sass. Here’s a breakdown of what will be included in your Gulp file:
- Task for compiling Sass: This task will take your SCSS files and compile them into CSS, placing the compiled files in the appropriate directory.
- Task for live reloading: This task will watch for changes to your SCSS, CSS, and PHP files. If any of these files change, BrowserSync will refresh the browser automatically.
const gulp = require('gulp');
const browserSync = require('browser-sync').create();
const sass = require('gulp-sass')(require('sass'));
// Compile SCSS into CSS
gulp.task('sass', function() {
return gulp.src('./style.scss')
.pipe(sass().on('error', sass.logError))
.pipe(gulp.dest('.'))
.pipe(browserSync.stream());
});
// Watch for changes and refresh browser
gulp.task('serve', function() {
browserSync.init({
proxy: "https://yourwordpresssite.test/", // Adjust to your local WordPress site URL
});
gulp.watch('./path/to/your/partials/*.scss', gulp.series('sass')); // Watch SCSS files for changes, and recompile css files when they do
//gulp.watch('**/*.php').on('change', browserSync.reload); // Uncomment if you want to reload on PHP files changes
//gulp.watch('./*.css').on('change', browserSync.reload); // Uncomment if you want to reload CSS files for changes
});
// Default Gulp task
gulp.task('default', gulp.series('sass', 'serve'));
Make sure to configure your gulpfile.js to ensure that it watches all SCSS files, including partials, and that it only compiles your main SCSS file (e.g., style.scss).
Running Gulp and Live Reloading
Once your gulpfile.js
is set up, you’re ready to start using live reloading. To start this, run Gulp in your terminal. Run the following command inside the theme directory:
gulp
This command will start the Gulp tasks and begin watching for file changes. As soon as you make a change to any of your SCSS, CSS, or PHP files, Gulp will automatically compile the SCSS (if necessary) and refresh your browser via BrowserSync. With Gulp running, anytime you modify a CSS, SCSS, or PHP file, the changes will immediately be reflected in the browser without the need to manually refresh the page. This can save you a lot of time, especially during design iterations.
By setting up live reloading, you can streamline your WordPress theme development process, making it faster and more efficient. Instead of constantly refreshing the browser, you can focus on coding while Gulp and BrowserSync handle the rest. I hope this helped you save some time in theme development. If you have any question, feel free to leave a comment below and I will get back to you as soon as I can.